P13-M01
【UNITY】タワーディフェンス2(自動制御)
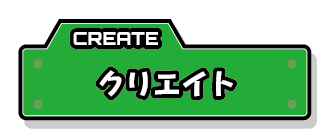
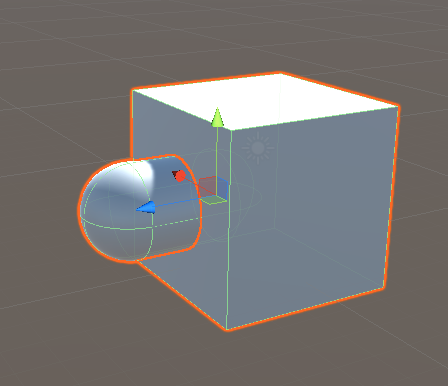
今回はタワーが自動で「敵」を攻撃するようにしよう!!
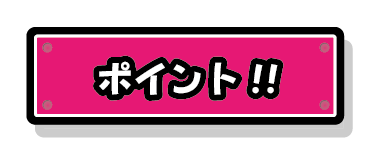
今回することは
1.タワーを一定間隔で弾を発射させる
2.最も近い「敵」を探し、その「敵」の方を向く
1
フィールドを整えよう
タワーディフェンスでよくあるような一直線のNavMeshを作成してみよう。Blenderなどのモデリングソフトを使って3Dデータを作ろう。
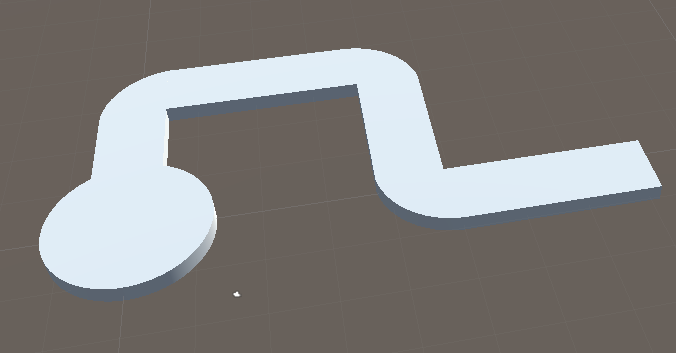
2
タワーから「弾」を一定間隔で発射する
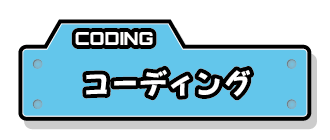
1.フリッパーで使う変数を定義
2.フリッパーで使う変数の初期化
3.フリッパーの開け閉めを制御するメソッドを追加
4.Updateメソッド内に、左矢印キーで左フリッパーを動かし、右矢印キーで右フリッパーを動かす処理を追加
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
using System.Collections; | |
using System.Collections.Generic; | |
using UnityEngine; | |
public class ShootingV2 : MonoBehaviour { | |
// 弾のGameObject | |
public GameObject bullet; | |
// 弾丸発射点のGameObjectのTransfom | |
public Transform muzzle; | |
public float speed = 10000; // 弾丸の速度 | |
public float timeOut = 20; // 時間を打つ間隔を指定 | |
private float timeElapsed; //経過時間を格納 | |
void Start() | |
{ | |
} | |
void Update() | |
{ | |
timeElapsed += Time.deltaTime; | |
if (timeElapsed >= timeOut) | |
{ | |
// 弾丸の複製(プレファブした弾GObjectを) | |
GameObject bullets = Instantiate(bullet) as GameObject; | |
// 変数:弾の力 | |
Vector3 force; | |
// 弾丸のはじめ位置を調整 | |
bullets.transform.position = muzzle.position; | |
// foceを計算する | |
force = this.gameObject.transform.forward * speed; | |
// Rigidbodyに力を加える | |
bullets.GetComponent<Rigidbody>().AddForce(force); | |
timeElapsed = 0.0f; | |
} | |
} | |
} |
3
タワーが一番近い「敵」の方を向くようにする
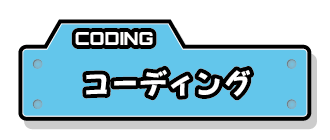
1.フリッパーで使う変数を定義
2.フリッパーで使う変数の初期化
3.フリッパーの開け閉めを制御するメソッドを追加
4.Updateメソッド内に、左矢印キーで左フリッパーを動かし、右矢印キーで右フリッパーを動かす処理を追加
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
using System.Collections; | |
using System.Collections.Generic; | |
using UnityEngine; | |
public class TowerContV2 : MonoBehaviour | |
{ | |
// 敵を検知するための変数 | |
GameObject[] Enemys; | |
GameObject nearestEnemy = null; | |
GameObject oldNearestEnemy = null; | |
float minDis = 1000f; | |
public float Range = 100f;//攻撃可能範囲 | |
// Use this for initialization | |
void Start() | |
{ | |
} | |
// Update is called once per frame | |
void Update() | |
{ | |
/************************ | |
* 一番近い敵を検知 | |
************************/ | |
minDis = 1000f; | |
// FindGameObjectsWithTagでタグ名からEnemyを検索しGameObject(配列)に格納 | |
Enemys = GameObject.FindGameObjectsWithTag("Enemy"); | |
// タグ名(Enemy)のゲームオブジェクト全部に対して順番に処理をしていく | |
foreach (GameObject enemy in Enemys) | |
{ | |
float disWk = Vector3.Distance(transform.position, enemy.transform.position); | |
if (disWk <= Range && disWk < minDis) | |
{ | |
minDis = disWk; | |
nearestEnemy = enemy; | |
} | |
} | |
// Enemyが更新されていなときは | |
if (nearestEnemy == oldNearestEnemy){ | |
// minDis = 1000f; | |
} | |
if (nearestEnemy != null ) { | |
oldNearestEnemy = nearestEnemy; | |
transform.rotation = Quaternion.Slerp(transform.rotation, Quaternion.LookRotation(nearestEnemy.transform.position - transform.position), 1.9f); | |
} | |
} | |
} |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
using System.Collections; | |
using System.Collections.Generic; | |
using UnityEngine; | |
using UnityEngine.AI; | |
public class GameCont : MonoBehaviour { | |
// 敵出現のための変数 | |
public GameObject Enemy; | |
public int EnemyNum; | |
int EnemyWk; | |
GameObject[] Enemys; | |
public Transform GolePoint; | |
NavMeshAgent agent; | |
// Use this for initialization | |
void Start () { | |
EnemyWk = 0; | |
} | |
// Update is called once per frame | |
void Update () { | |
/************************ | |
* 敵の出現をコントロール | |
************************/ | |
// 敵をタグで取得 | |
Enemys = GameObject.FindGameObjectsWithTag("Enemy"); | |
EnemyWk = Enemys.Length; | |
// 敵が出現する地点を指定 | |
Vector3 vec1 = new Vector3(-5.02f, 4.25f, -77.95f); | |
if (EnemyWk < EnemyNum) | |
{ | |
GameObject EnemyObj = Instantiate(Enemy, vec1, Quaternion.identity) as GameObject; | |
agent = EnemyObj.GetComponent<NavMeshAgent>(); | |
agent.SetDestination(GolePoint.position); | |
} | |
} | |
} |