P1-M02
【UNITY】タワーディフェンス3(回答編)
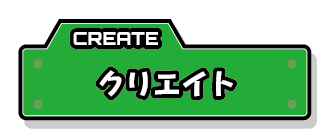
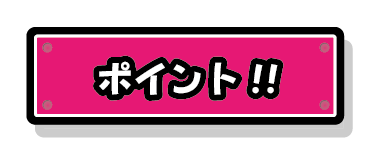
この回は、【UNITY】タワーディフェンス3(タワーの設置他)の課題3(タワーのおける数を制限してみよう)の回答案です。
1
出現しているオブジェクトの数の取得方法
クリックによって出現させてオブジェクトの数を取得する方法は主に2つあります。
1.オブジェクトの数を格納しておく変数を定義し、出現(もしくは削除)する時に変数を操作(+1,-1)をする。
2.オブジェクトにTag(タグ)を定義しておき、Tagでオブジェクトを取得しその数(Length)を使う。
【1のメリット】
1は操作がほぼプログラム内だけで終わり、簡単です。
【1のデメリット】
オブジェクトの出現、削除のプロセスが複雑になるとタイミングを間違えやすいなったり、プログラムが複雑になってしまう。
【2のメリット】
現在実際に出現しているオブジェクトをTagで取得して数を取得するため、考え方と処理が簡略的。将来の拡張の際にもバグが出にくい。
【2のデメリット】
プログラムの他タグ設定など多少操作が複雑。
2の方法でクリックで出現するオブジェクト数を制限する
まず「 Tower」オブジェクに設定しておくタグ(Tag)「タグ名:Tower」を設定する。
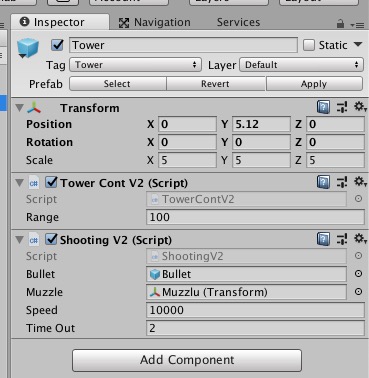
3
課題回答例
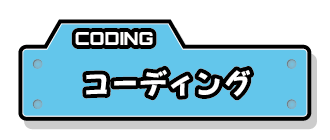
1.オブジェクトの出現最大可能数を格納する変数の定義(ヒエラルキーから定義できるように)
2.現在出現しているオブジェクト数を取得する
3.取得したオブジェクト数が出現最大可能数より小さければクリック時の処理を行う
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
using System.Collections; | |
using System.Collections.Generic; | |
using UnityEngine; | |
using UnityEngine.AI; | |
public class GameCont : MonoBehaviour { | |
// 敵出現のための変数 | |
public GameObject Enemy; | |
public int EnemyNum; | |
int EnemyWk; | |
GameObject[] Enemys; | |
public Transform GolePoint; | |
NavMeshAgent agent; | |
/******************** | |
* クリックによるオブジェクト配置処理のための変数定義 | |
*********************/ | |
// ポンタ表示のためのオブジェクト | |
public GameObject pointerOBJ; | |
// ディフェンダーオブジェクト | |
public GameObject defenderOBJ; | |
//クリックによって出現させるオブジェクトの数のコントロール | |
public int ObjectNum;//出現させるオブジェクトの最高値を格納 | |
int ObjectWk;//現在のオブジェクト数を格納 | |
GameObject[] GO; // クリックしておいたゲームオブジェクトを格納 | |
// Use this for initialization | |
void Start () { | |
EnemyWk = 0; | |
ObjectWk = 0; | |
} | |
// Update is called once per frame | |
void Update () { | |
/************************ | |
* 敵の出現をコントロール | |
************************/ | |
// 敵をタグで取得 | |
Enemys = GameObject.FindGameObjectsWithTag("Enemy"); | |
EnemyWk = Enemys.Length; | |
// 敵が出現する地点を指定 | |
Vector3 vec1 = new Vector3(-5.02f, 4.25f, -77.95f); | |
if (EnemyWk < EnemyNum) | |
{ | |
GameObject EnemyObj = Instantiate(Enemy, vec1, Quaternion.identity) as GameObject; | |
agent = EnemyObj.GetComponent<NavMeshAgent>(); | |
agent.SetDestination(GolePoint.position); | |
} | |
/************************ | |
* レイキャスト | |
* マウスがクリックされた位置(オブジェクト)を表示 | |
************************/ | |
//メインカメラ上のマウスカーソルのある位置からRayを飛ばす | |
Ray ray = Camera.main.ScreenPointToRay(Input.mousePosition); | |
RaycastHit hit; | |
// | |
//すでに出現させたオブジェクトの数を取得する | |
//すでに出現させたオブジェクトを取得し、変数(GO)に格納 | |
// | |
GO = GameObject.FindGameObjectsWithTag("Tower"); | |
// 変数(GO)の個数を変数ObjectWkに格納 | |
ObjectWk = GO.Length; | |
if (Physics.Raycast(ray, out hit, Mathf.Infinity)) | |
{ | |
//Rayが当たるオブジェクトがあった場合はそのオブジェクト名をログに表示 | |
Debug.Log("Ray:" + hit.collider.gameObject.name); | |
pointerOBJ.transform.position = hit.point; | |
if (Input.GetMouseButtonDown(0)) | |
{ | |
// オブジェクト | |
if (ObjectWk < ObjectNum) { | |
GameObject Defender = Instantiate(defenderOBJ, hit.point, Quaternion.identity) as GameObject; | |
} | |
} | |
} | |
} | |
} |