プレイグランドを改造する
Improvement Playground
今回はUNITYの練習用アセットPlaygroundの改良をしてみましょう。今回は時間制限機能をつけてみましょう。
LEVEL
開発環境
UNITY (2D)
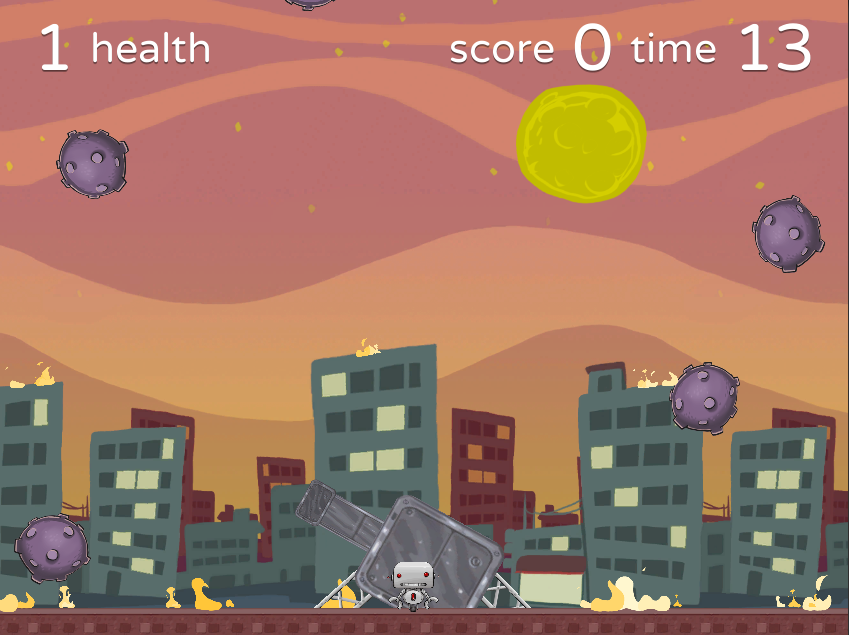
対応デバイス:PC方向変更:WADS レーザー発射:スペース
プレイグランドに時間制限機能をつける
プレイグランドは、色々なアセットで簡単な操作でゲームを作れるようになっていますが、通常のUnityプロジェクトと同じように色々なオリジナル機能を追加することができます。今回は、時間制限の機能をつけてみましょう。
編集しよう
変更箇所
【プレファブ】Prefabs
・Userinterface
【スクリプト】Scripts
・UIScript
・UIScriptInspector
プレファブの変更
Userinterfaceプレファブを編集していきます。「Time Up」を表示するためのPanel(パネル)をつくります。
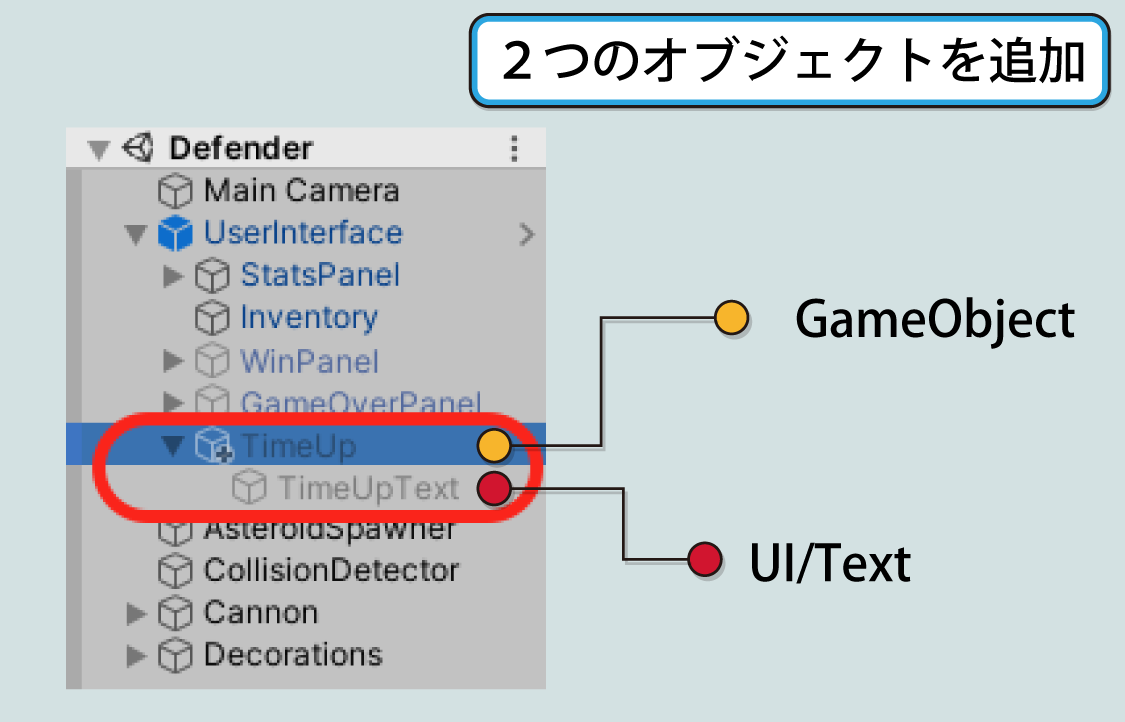
ゲームオブジェクト「TimeUp」の設定
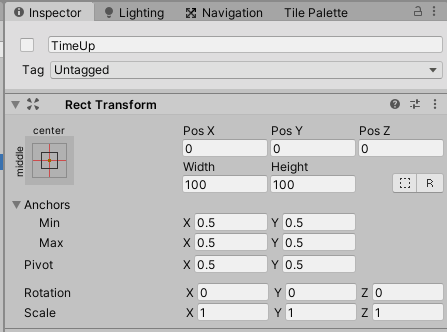
UI TEXT「TimeUpText」の設定
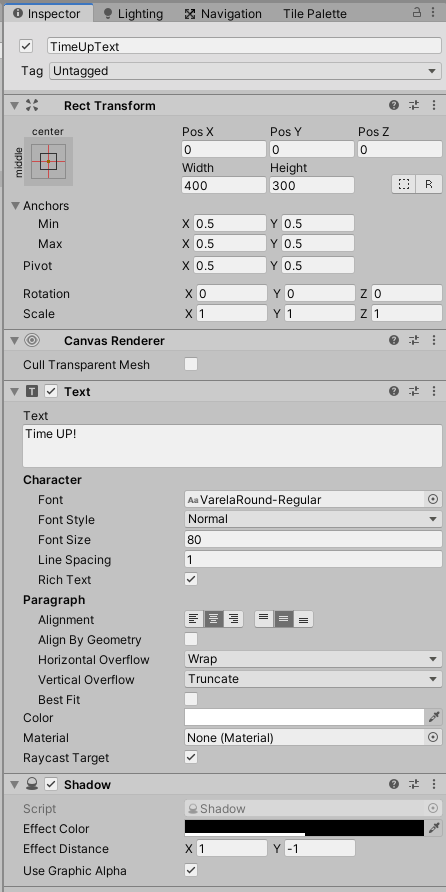
スクリプトの変更
UIScript.cs
時間を計測するための各種変数を定義して、Update()メソッドを追加して、そのなかで時間を計測させています。
変更箇所は「改良のための追加..」を書かれています。
UIScriptInspector.cs
このスクリプトは、UIScriptのインスペクターの表示を編集するためのスクリプトです。ですから、このスクリプトにUIScriptインスペクタで今回追加した変数をインスペクタに表示するコードを追加します。変更箇所は「改良のための追加..」を書かれています。
コードを書くとUserInterfaceのインスペクタに以下のように変数が追加されますので、該当のGameObjectをアタッチしてください。Game Time変数に0を入力するとカウントアップしていきます。また、0より大きな数字をいれるとカウントダウンして、0になると「タイムアップ」と表示されうます。
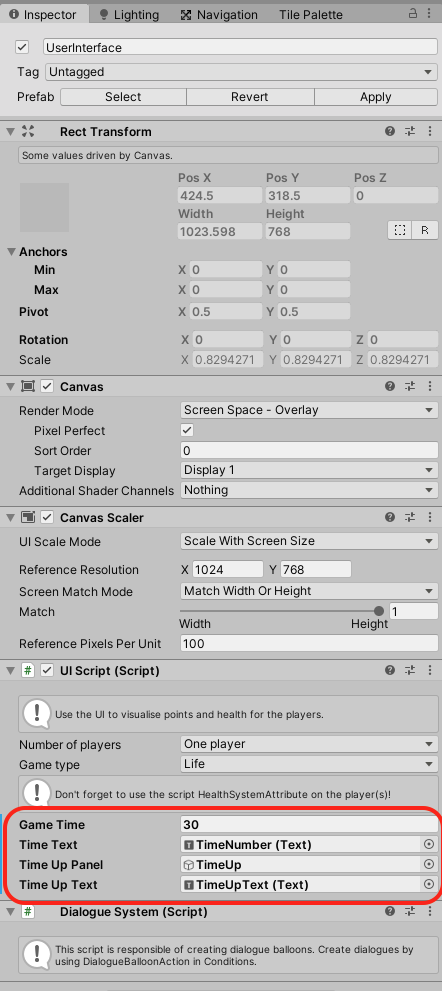
これで改良のおわりです。下のように時間表示は追加されるとおもいます。
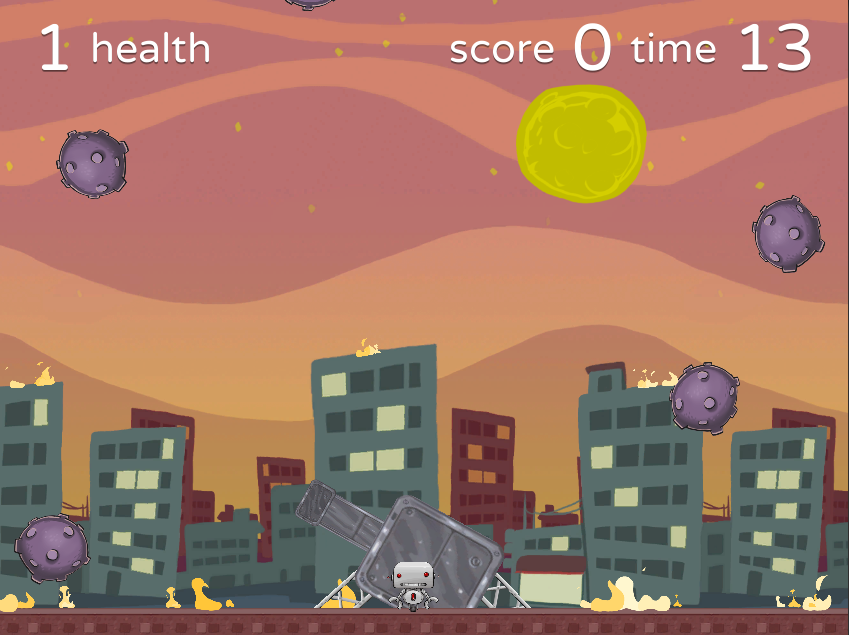