Portfolio
受賞作品「SPACE FIGHTER」
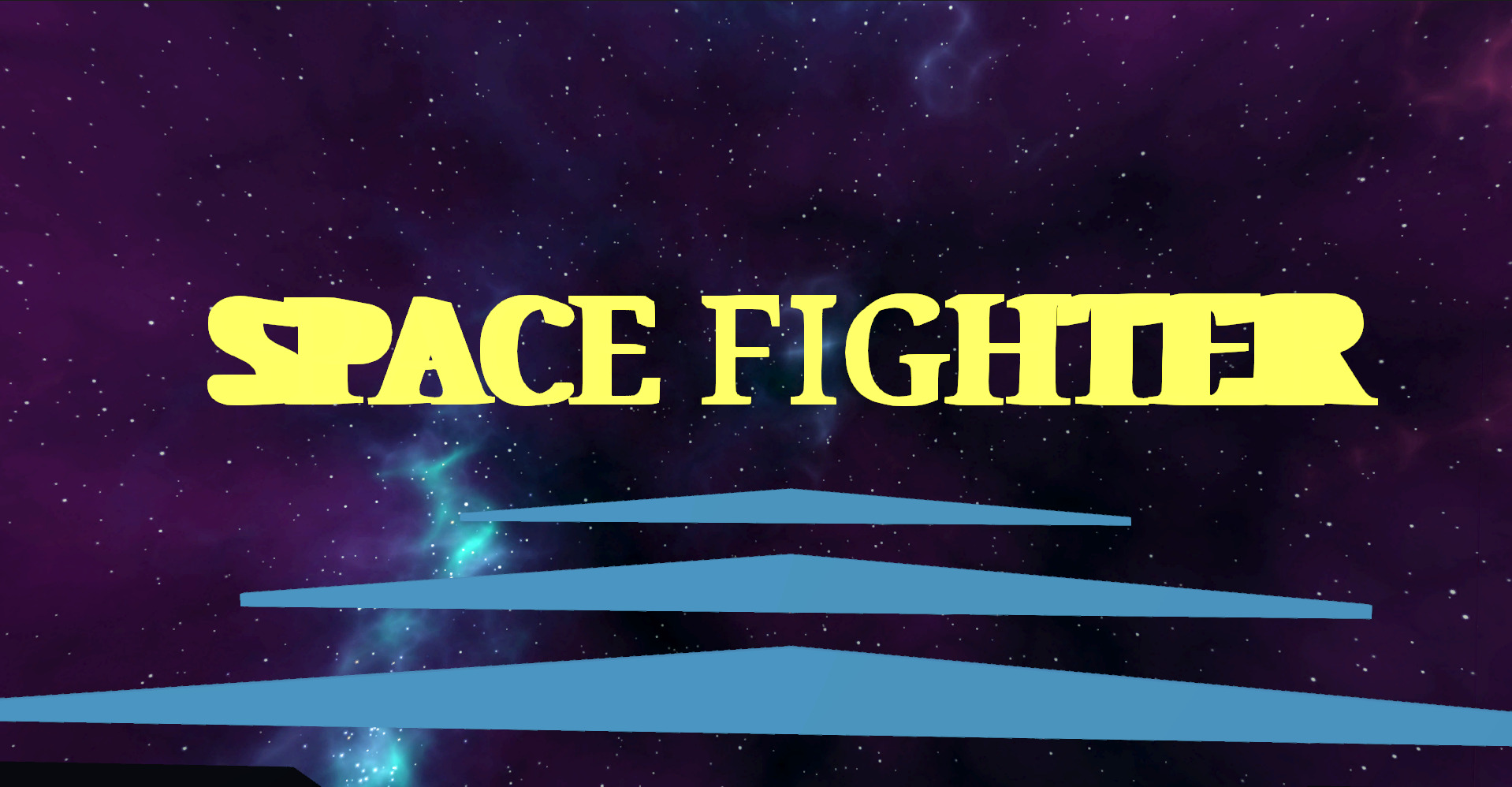
ゲーム紹介
制作者: 河方(中3)
オープニングエリアからパイロットを操作し、その後シューティング画面へと進む構成になっています。オープニングから細部にこだわった作り込みを目指しており、プレイヤーを引き込む演出や操作性を重視しています。
プレイ動画
面紹介
この3Dシューティングゲームは、オープニングからプレイヤーをゲームの世界に引き込むことを目指し、特にカメラワークにこだわって作り上げています。パイロットを操作しながら展開するオープニングエリアは、物語の序章としての臨場感を演出。シューティング画面では、操作のシビアさがあり調整が必要と考える。
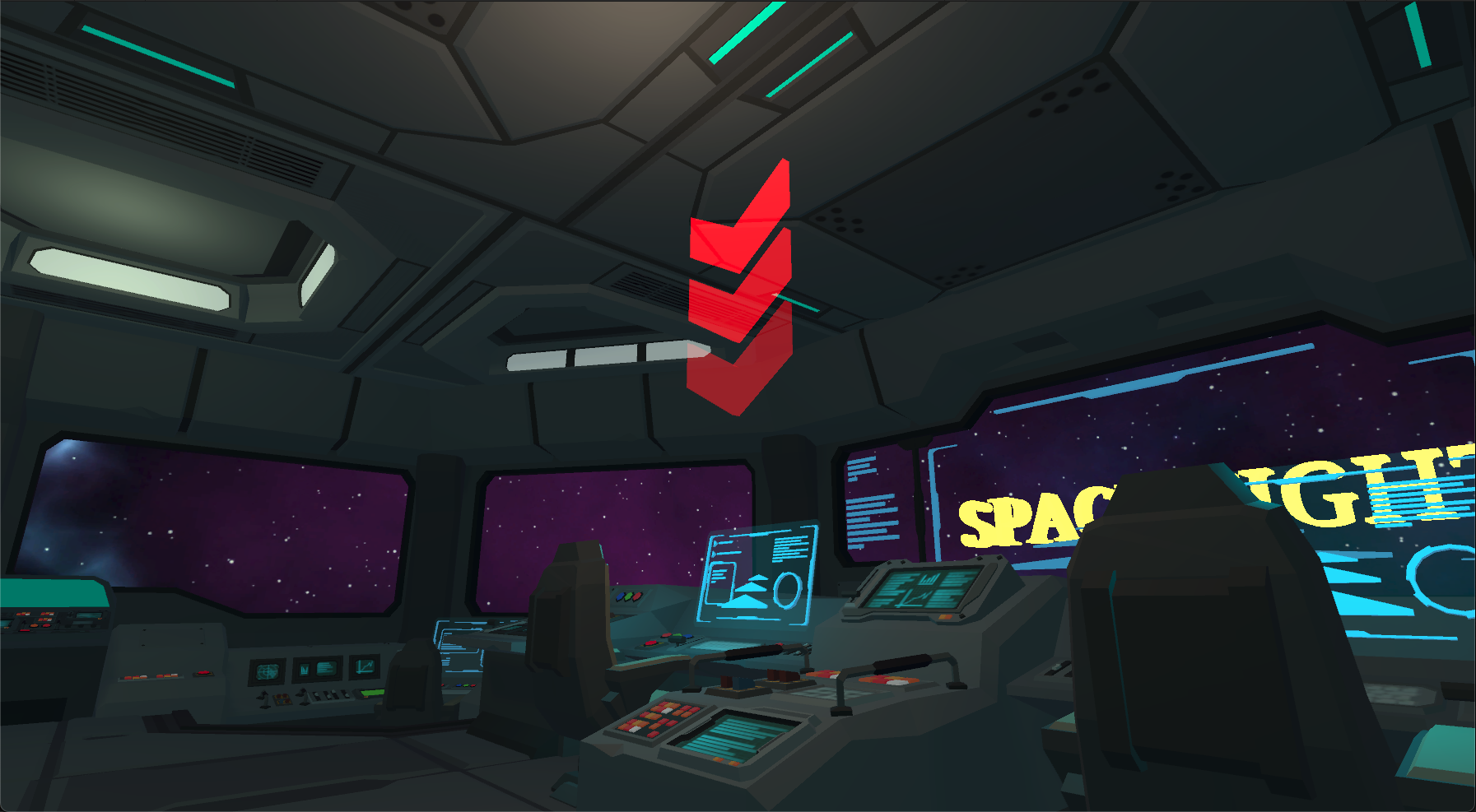
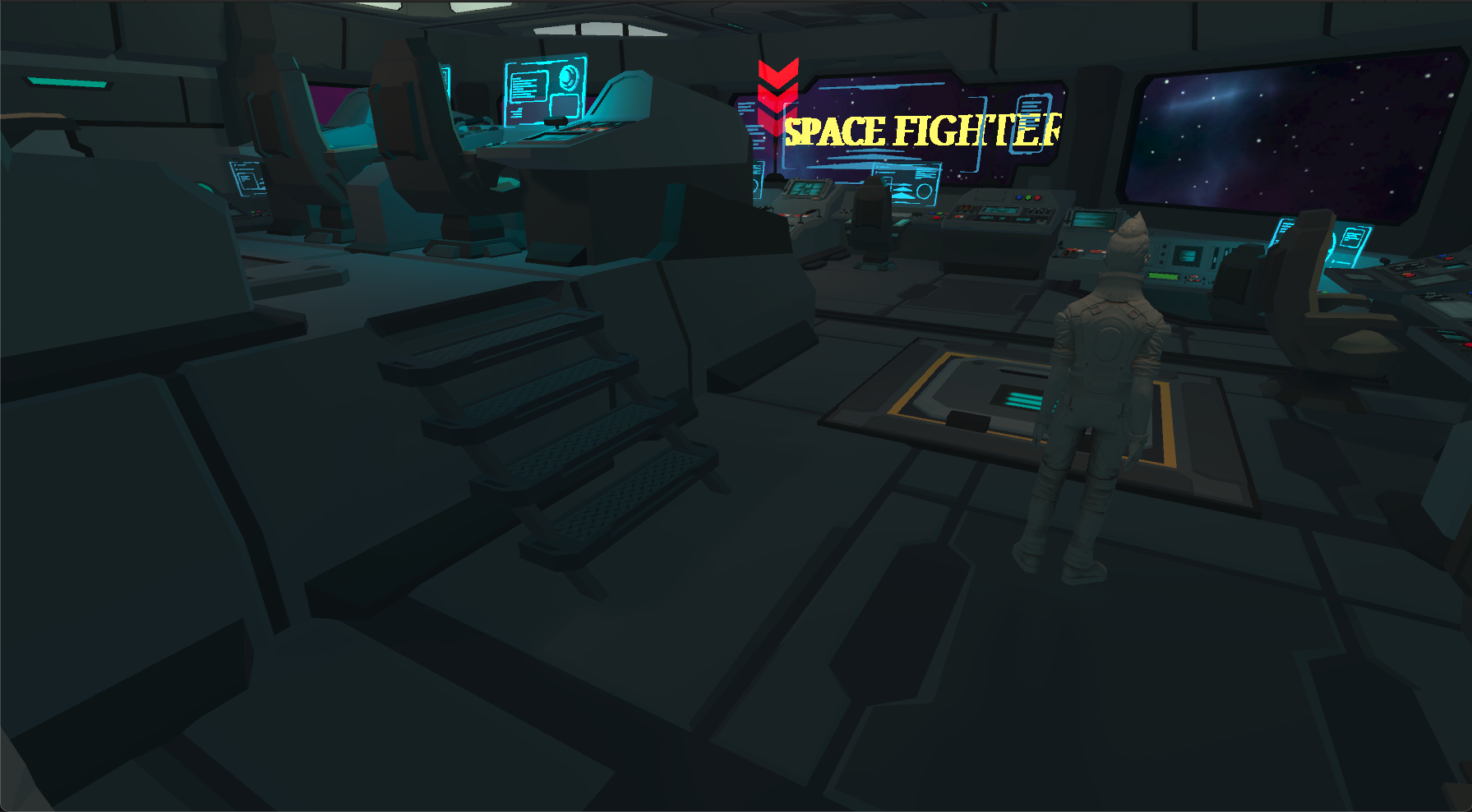
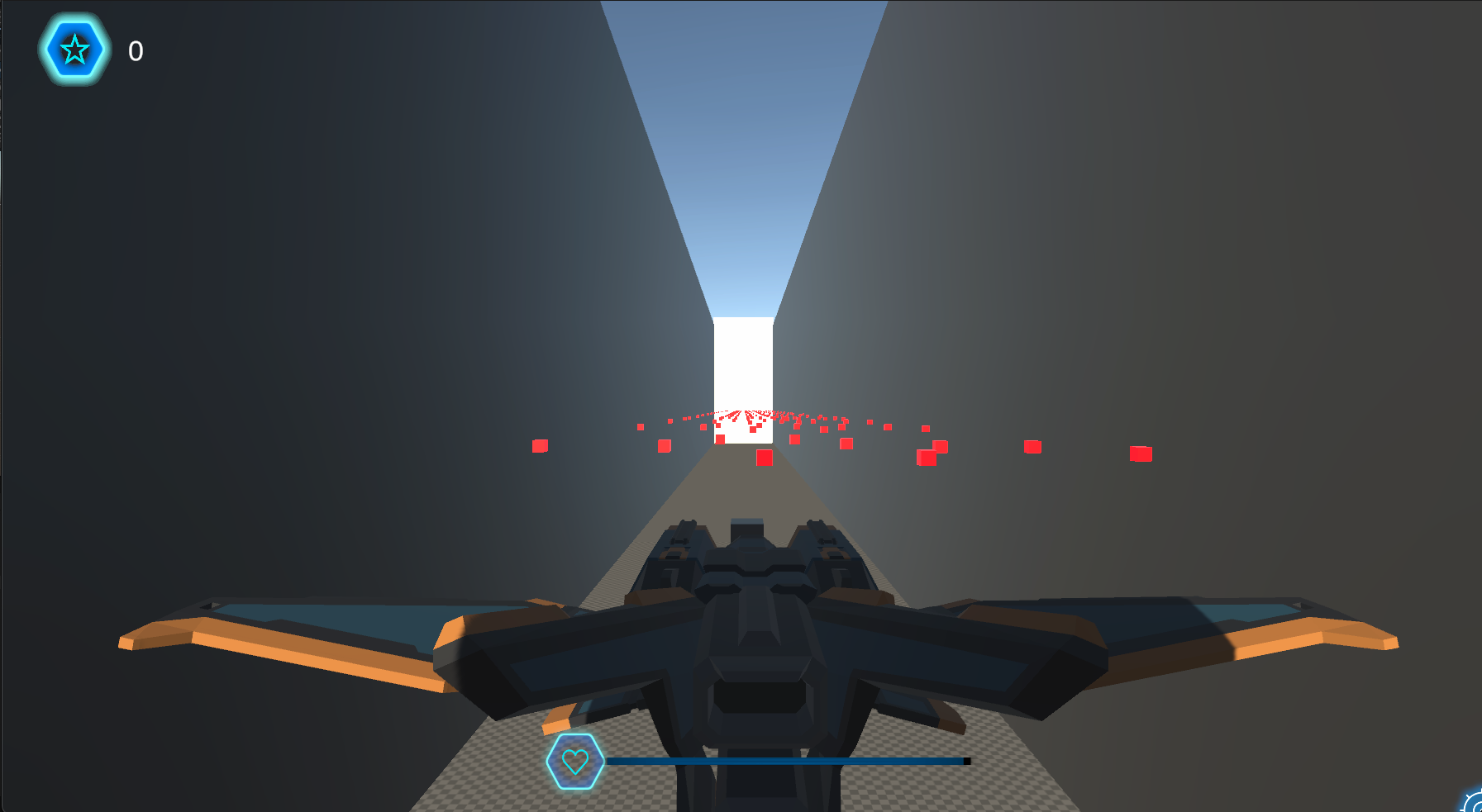
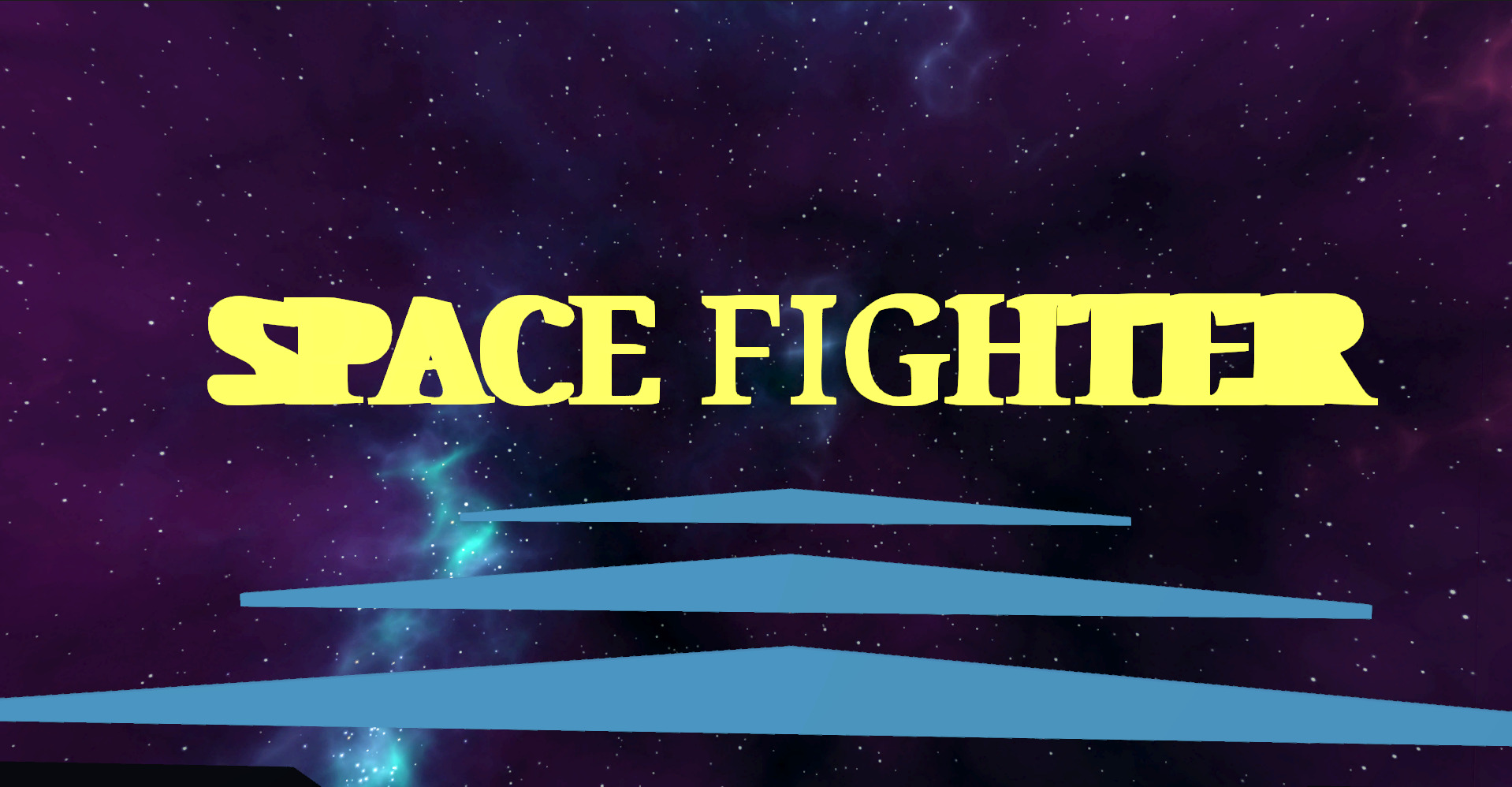
プログラミング
プログラムの一部を紹介いたします。
●弾をコントロールするプログラム
using UnityEngine;
public class FireBullet : MonoBehaviour
{
[SerializeField]
[Tooltip("弾の発射場所")]
private GameObject firingPoint;
[SerializeField]
[Tooltip("弾")]
private GameObject bullet;
[SerializeField]
[Tooltip("弾")]
private GameObject Sparkle;
[SerializeField]
[Tooltip("弾の速さ")]
private float speed = 10000f;
// Update is called once per frame
void Update()
{
RaycastHit hit;
Ray ray = Camera.main.ScreenPointToRay(Input.mousePosition);
// スペースキーが押されたかを判定
if (Input.GetMouseButtonDown(0))
{
if (Physics.Raycast(ray, out hit, Mathf.Infinity))
{
// 弾を発射する場所を取得
Vector3 bulletPosition = firingPoint.transform.position;
Vector3 direction = hit.point - this.transform.position;
Vector3 directionWk = direction.normalized;
firingPoint.transform.LookAt(hit.point);
// 上で取得した場所に、"bullet"のPrefabを出現させる。Bulletの向きはMuzzleのローカル値と同じにする(3つ目の引数)
GameObject newBullet = Instantiate(bullet, bulletPosition, firingPoint.transform.rotation*Quaternion.Euler(90,0,0));
GameObject newSparkle = Instantiate(Sparkle, bulletPosition, firingPoint.transform.rotation*Quaternion.Euler(90,0,0),newBullet.transform);
// 出現させた弾のup(Y軸方向)を取得(MuzzleのローカルY軸方向のこと)
//Vector3 direction = newBullet.transform.forward;
Debug.Log(directionWk * speed + ",direction" + direction);
// 弾の発射方向にnewBallのY方向(ローカル座標)を入れ、弾オブジェクトのrigidbodyに衝撃力を加える
newBullet.GetComponent().AddForce(directionWk * speed, ForceMode.Impulse);
// 出現させた弾の名前を"bullet"に変更
newBullet.name = bullet.name;
Destroy(newBullet, 5f);
}
}
}
}
●プレイヤーをコントロールするプログラム
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class Airplane : MonoBehaviour
{
private float miuTurnInputValue;
private Rigidbody miuRb;
private float miuNoseInputValue;
private void Start()
{
// Debug.Log ("Start");
miuRb = GetComponent();
}
void Update()
{
//Debug.Log("hello");
// 前進は自動
transform.Translate(0f, 0f, 7f * Time.deltaTime);
// 旋回
miuTurnInputValue = Input.GetAxis("Horizontal");
float turn = miuTurnInputValue * 100 * Time.deltaTime;
Quaternion turnRotation = Quaternion.Euler(0, turn, 0);
miuRb.MoveRotation(miuRb.rotation * turnRotation);
// Debug.Log("good bye");
// 機首(上昇、下降)
miuNoseInputValue = Input.GetAxis("Vertical");
float noseTurn = miuNoseInputValue * 30 * Time.deltaTime;
Quaternion turnNoseRotation = Quaternion.Euler(noseTurn, 0, 0);
miuRb.MoveRotation(miuRb.rotation * turnNoseRotation);
}
}
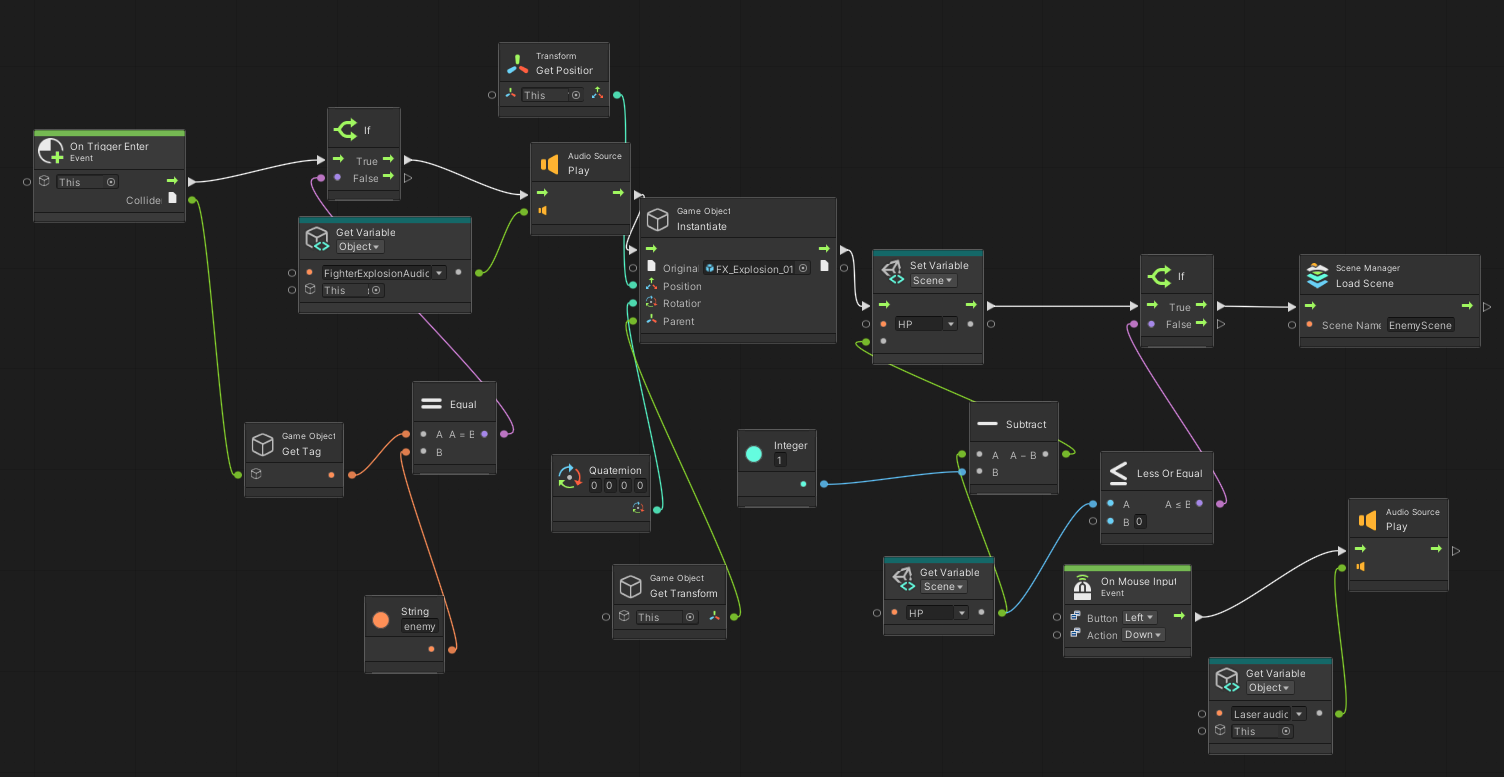
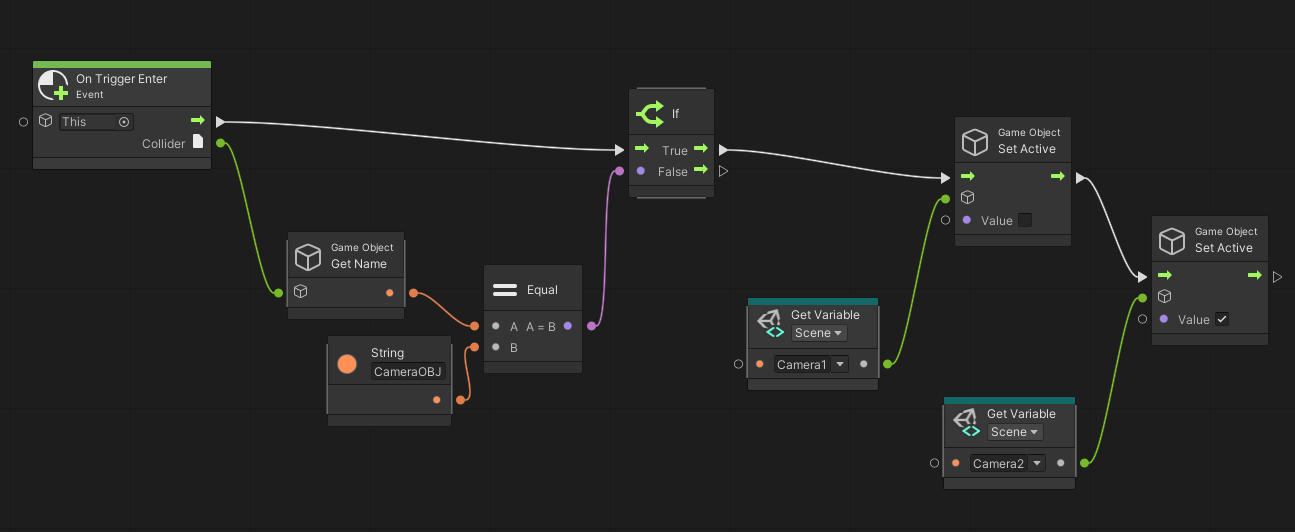
●カメラワークを操作するドリー設定
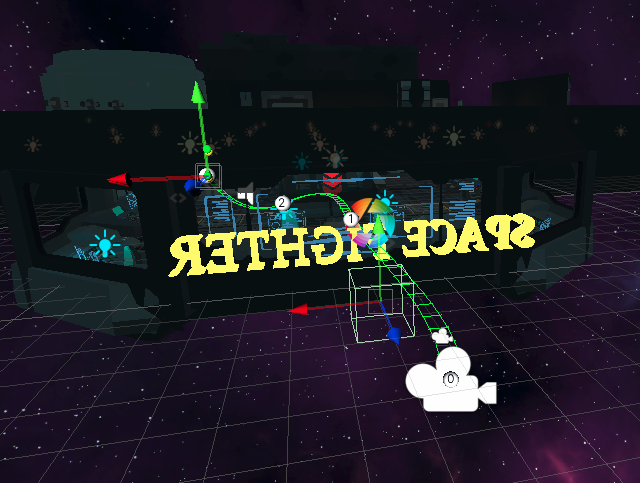
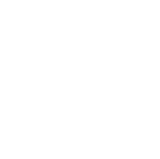
DIGILAB edutainment Company
デジラボは、プログラミング教育をはじめとする多彩なSTEAM教育の開発と普及を支援しています。
Copyright © 2025 Digilab. All Rights Reserved.